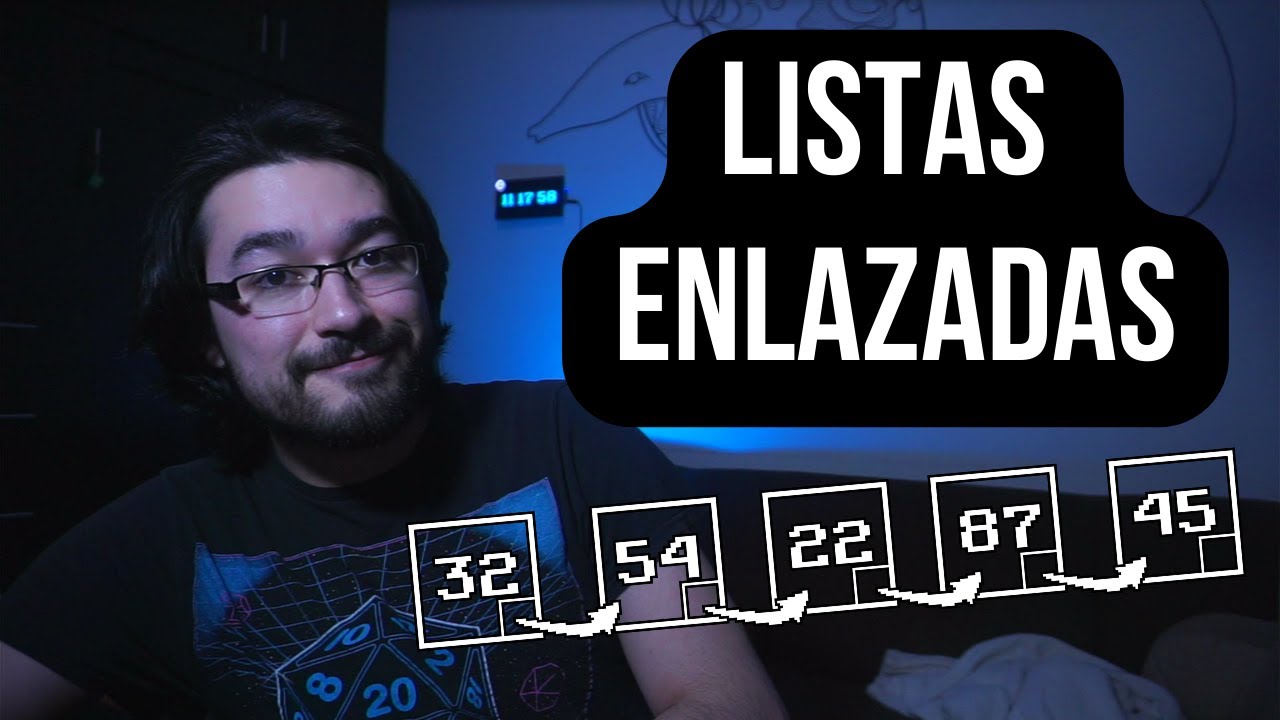
How do you find whether there is a loop in the linked list or not how do you find the starting node of the loop?
To detect the start of the loop, consider the below algorithm. Step 1: Move ‘S’ to the start of the list, but ‘F’ would remain point to node 3. Step 2: Move ‘S’ and ‘F’ forward one node at a time until they meet. Step 3: The node where they meet is the start of the loop.
- Q. How do you find the loop in a linked list?
- Q. What is the best way to detect a cycle in a linked list?
- Q. Is it possible to find a loop in a linked list time complexity?
- Q. Does a linked list have a cycle?
- Q. How many cycles can a linked list have?
- Q. How do you find the length of a singly linked list?
- Q. Can you find a loop in a linked list Mcq?
- Q. What is cycle in a linked list?
- Q. Does linked list have cycle?
- Q. What is a linked list data structure?
- Q. What is a linked list in C programming?
- Q. What is linked list implementation?
- Q. How do you know if a singly linked list is cyclic?
- Q. How do you check if the linked list contains a loop in Java?
- Q. How do you check if a given linked list contains a cycle How do you find the starting node of the cycle solution?
- Q. Is it possible to find a loop in a linked list?
- Q. How do you know if a linked list is circular?
- Q. How would you check if a linked list has cycles?
- Q. How do you determine if a linked list is circular?
- Q. How do you loop a linked list?
Q. How do you find the loop in a linked list?
Approach: This solution requires modifications to the basic linked list data structure.
- Have a visited flag with each node.
- Traverse the linked list and keep marking visited nodes.
- If you see a visited node again then there is a loop.
Q. What is the best way to detect a cycle in a linked list?
You can make use of Floyd’s cycle-finding algorithm, also known as tortoise and hare algorithm. The idea is to have two references to the list and move them at different speeds. Move one forward by 1 node and the other by 2 nodes. If the linked list has a loop they will definitely meet.
Q. Is it possible to find a loop in a linked list time complexity?
The normal scenario to find the loop in the linked list is to move a pointer once and move other pointer two times. If they meet,there is a loop in the linked list.
Q. Does a linked list have a cycle?
There is a cycle in a linked list if there is some node in the list that can be reached again by continuously following the next pointer. Internally, pos is used to denote the index of the node that tail’s next pointer is connected to.
Q. How many cycles can a linked list have?
Note that we can have only one cycle inside a list. Besides, the cause of the cycle will always be that the last node is pointing to a node inside the list because each node points to exactly one node.
Q. How do you find the length of a singly linked list?
Algorithm:
- Find the common point in the loop by using the Floyd’s Cycle detection algorithm.
- Store the pointer in a temporary variable and keep a count = 0.
- Traverse the linked list until the same node is reached again and increase the count while moving to next node.
- Print the count as length of loop.
Q. Can you find a loop in a linked list Mcq?
Explanation: Loop through the list to get into position one behind the actual position given. Elements are accessed sequentially in linked list. Random access of elements is not an applications of linked list. 9.
Q. What is cycle in a linked list?
A cycle occurs when a node’s next points back to a previous node in the list. The linked list is no longer linear with a beginning and end—instead, it cycles through a loop of nodes.
Q. Does linked list have cycle?
There is a cycle in a linked list if there is some node in the list that can be reached again by continuously following the next pointer.
Q. What is a linked list data structure?
Recent Articles on Linked List. A linked list is a linear data structure, in which the elements are not stored at contiguous memory locations. The elements in a linked list are linked using pointers as shown in the below image: In simple words, a linked list consists of nodes where each node contains a data field and a reference(link) to the next node in the list.
Q. What is a linked list in C programming?
Linked List Program in C. A linked list is a sequence of data structures, which are connected together via links. Linked List is a sequence of links which contains items. Each link contains a connection to another link. Linked list is the second most-used data structure after array.
Q. What is linked list implementation?
Singly linked list implementation. Singly Linked Lists are a type of data structure. It is a type of list. In a singly linked list each node in the list stores the contents of the node and a pointer or reference to the next node in the list. It does not store any pointer or reference to the previous node.
Q. How do you know if a singly linked list is cyclic?
How might we identify whether the given linked list is circular?
- If any node seems to be pointing towards the head or starting node then the linked list is circular.
- If no node is pointing to null.
Q. How do you check if the linked list contains a loop in Java?
Move fastPtr by two nodes and slowPtr by one node in each iteration. If fastPtr and slowPtr meet at some iteration , then there is a loop in linkedlist. If fastPtr reaches to the end of linkedlist without meeting slow pointer then there is no loop in linkedlist (i.e fastPtr->next or fastPtr->next->next become null)
Q. How do you check if a given linked list contains a cycle How do you find the starting node of the cycle solution?
- # Function to detect a cycle in a linked list using hashing.
- def detectCycle(head): curr = head.
- s = set() # traverse the list.
- while curr:
- if curr in s:
- # insert the current node into the set.
- # move to the next node.
- # we reach here if the list does not contain any cycle.
Q. Is it possible to find a loop in a linked list?
A loop exists in a LinkedList when no NULL is reached as we traverse throughout the LinkedList. So in order to detect whether a LinkedList has a loop or not, we can traverse through the LinkedList and add each Node to the HashSet of visited notes if it’s been visited for the first item.
Q. How do you know if a linked list is circular?
To check whether the linked list is circular or not, we will store the header node into some other variable, then traverse the list, if we get null at the next part of any node, then that is not circular, otherwise we will check the next node is same as the stored node or not, if so then that is circular.
Q. How would you check if a linked list has cycles?
Q. How do you determine if a linked list is circular?
Q. How do you loop a linked list?
- To iterate the LinkedList using the iterator we first create an iterator to the current list and keep on printing the next element using the next() method until the next element exists inside the LinkedList.
- We check if the LinkedList contains the next element using the hasNext() method.
Las listas enlazadas son una estructura de datos básica en la programación. En el video explico cuáles son sus características e implementación usando Java, …
No Comments