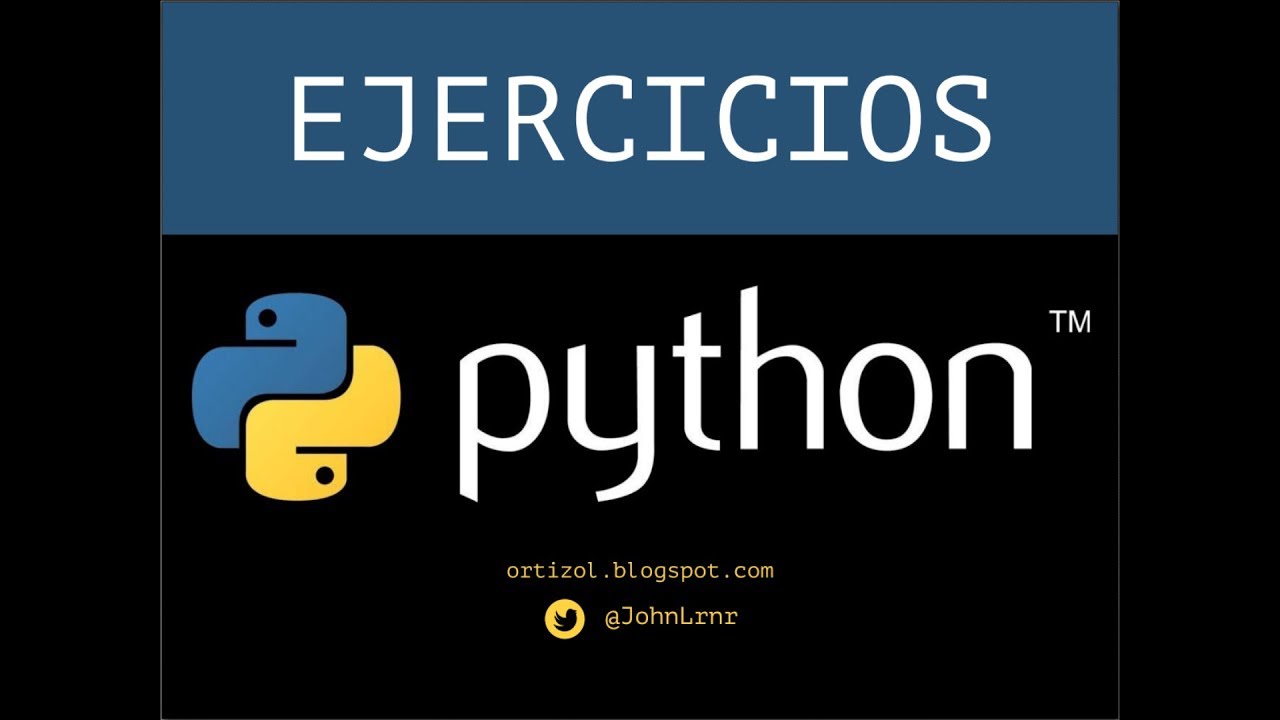
How do you find the characters that are being repeated in a string?
An efficient solution is to use Hashing to solve this in O(N) time on average.
- Q. How do you repeat in RegEx?
- Q. How do you find a regular expression in a string?
- Q. How do I find a repeated character in a string in SQL?
- Q. How do you find the first repeated and non repeated character in a string?
- Q. How do I find a repeated character in a string python?
- Q. How to check for repeating characters in regex?
- Q. How to find a repeated substring in a string?
- Q. How to find the first repeated character in a string?
- Q. How to check for duplicate words in regex?
- Q. How do you remove duplicate characters from a string in Python?
- Q. How to get rid of repeated characters in a string?
- Q. How to remove duplicate characters from a string?
- Q. Is there a limit to how many repeated characters in a string?
- Q. When to call recursion on a empty string?
- Q. How do you find repeats in a string python?
- Q. How do I find the most repeated characters in a string in python?
- Q. How do you repeat a string 10 times in python?
- Q. Why is * called string repetition operator?
- Q. How to find non repeating characters in a string?
- Q. How to check if a string repeats itself in Python?
- Q. How to print a string of repeated characters in Python?
- Q. How to find the largest repeating substring in Python?
- Create an empty hash.
- Scan each character of input string and insert values to each keys in the hash.
- When any character appears more than once, hash key value is increment by 1, and return the character.
Q. How do you repeat in RegEx?
A repeat is an expression that is repeated an arbitrary number of times. An expression followed by ‘*’ can be repeated any number of times, including zero. An expression followed by ‘+’ can be repeated any number of times, but at least once.
Q. How do you find a regular expression in a string?
With RegEx you can use pattern matching to search for particular strings of characters rather than constructing multiple, literal search queries….Thus, if you are searching for varying strings that all begin with NLRT, such as:
- NLRT-0381.
- NLRT-6334.
- NLRT-9167.
- The proper Relativity RegEx is: “##nlrt-/d{4}”.
Q. How do I find a repeated character in a string in SQL?
SQL Server: Count Number of Occurrences of a Character or Word in a String
- DECLARE @tosearch VARCHAR(MAX)=’In’
- SELECT (DATALENGTH(@string)-DATALENGTH(REPLACE(@string,@tosearch,”)))/DATALENGTH(@tosearch)
- AS OccurrenceCount.
Q. How do you find the first repeated and non repeated character in a string?
Step 1 : Define one HashMap called charCountMap with Character as key and Integer as value. This map will hold the characters and their count in the given string. Step 2 : Convert inputString to char array called strArray. Step 3 : Iterate through all chars of strArray and update their occurrences in charCountMap.
Q. How do I find a repeated character in a string python?
Python
- string = “Great responsibility”;
- print(“Duplicate characters in a given string: “);
- #Counts each character present in the string.
- for i in range(0, len(string)):
- count = 1;
- for j in range(i+1, len(string)):
- if(string[i] == string[j] and string[i] != ‘ ‘):
- count = count + 1;
Q. How to check for repeating characters in regex?
The problem I am having is how to alter it so that it picks up if just the same character is entered a number of times (e.g. 17 x’s). In order to do that you’d need to use the catching parentheses. It’s a concept in which whatever you surround with parentheses will be captured into the memory of the regular expression parser.
Q. How to find a repeated substring in a string?
If the length of the input string is some multiple lps and last character of the string matches with the last character of the lps string. Then we have a substring (which is lps itself) of the input string which when repeated (or appended) a certain number of times gives the input string itself.
Q. How to find the first repeated character in a string?
When any character appears more than once, hash key value is increment by 1, and return the character. Below image is a dry run of the above approach: Below is the implementation of the above approach:
Q. How to check for duplicate words in regex?
Then, it matches all full words ( (//b//S+//b) ), followed by an end of string ( $) or a number of spaces ( //s+ ), the whole repeated more than once. Use this in case you want case-insensitive checking for duplicate words. Thanks for contributing an answer to Stack Overflow!
Q. How do you remove duplicate characters from a string in Python?
Program to remove duplicate characters from the given string using Python
- from collections import OrderedDict.
- def rem_dup_unorder(x):
- # Unordered output.
- output = ”. join(set(x))
- print(“String after removing duplicates using set() “, output)
- def rem_dup_ord(x):
- # Ordered output.
- output = ”. join(OrderedDict.
Q. How to get rid of repeated characters in a string?
Alternatively, repeated characters can be eliminated by sorting our input string to group duplicates. In order to do that, we have to convert the string to a char array and sort it using the Arrays.sort method. Finally, we’ll iterate over the sorted char array.
Q. How to remove duplicate characters from a string?
The naive approach to removing duplicates from a string simply involves looping over the input and using the indexOf method to check whether the current character already exists in the resulting string: Time Complexity: O (n * n) – for each character, the indexOf method runs through the remaining string
Q. Is there a limit to how many repeated characters in a string?
Something like “Too many repeated characters.” If the latter is acceptable, @” (//w)//1 {2}” should match characters of 3 or more (interpreted as “repeated” two or more times). Edit: As @Piskvor pointed out, this will match on exactly 3 characters.
Q. When to call recursion on a empty string?
If the string is empty, return. Else compare the adjacent characters of the string. If they are same then shift the characters one by one to the left. Call recursion on string S If they not same then call recursion from S+1 string. The recursion tree for the string S = aabcca is shown below. Below is the implementation of the above approach:
Q. How do you find repeats in a string python?
You can do it by repeating the substring a certain number of times and testing if it is equal to the original string. Start by building a prefix array. Loop through it in reverse and stop the first time you find something that’s repeated in your string (that is, it has a str. count()>1 .
Q. How do I find the most repeated characters in a string in python?
We find maximum occurring character by using max() on values. The most suggested method that could be used to find all occurrences is this method, this actually gets all element frequency and could also be used to print single element frequency if required. We find maximum occurring character by using max() on values.
Q. How do you repeat a string 10 times in python?
Use a function to repeat a string to a specific length
- Find the number of string repetitions needed for the target number of characters.
- Use * to repeat the string.
- Use string slicing to get the target number of characters from the repeated string.
- Return the string.
Q. Why is * called string repetition operator?
We are accustomed to using the * symbol to represent multiplication, but when the operand on the left side of the * is a tuple, it becomes the repetition operator. The repetition operator makes multiple copies of a tuple and joins them all together. Tuples can be created using the repetition operator, *.
Q. How to find non repeating characters in a string?
In this python program, we will find unique elements or nonrepeating elements of the string. Step 1:- Start. Step 2:- Take user input. Step 3:- Start iterating through string. Step 4:- Initialize count variable. Step 5:- Again start iterating through same string. Step 6:- Increment count variable as character is found in string.
Q. How to check if a string repeats itself in Python?
While working with strings, many times, we can come across a use case in which we need to find if a string has in it the repeating substring, which repeats all over the string and thus making a multiple of the root substring. Let’s discuss certain ways in which we can get the root substring of string.
Q. How to print a string of repeated characters in Python?
Given a string of characters. Write python code to print the repeated characters This is a typical dictionary based problem. Here is how to do that in Python. Thanks for visiting.
Q. How to find the largest repeating substring in Python?
It compares the first letter with every other until it finds a match, then saves it. Then it compares the first 2 letters with every other until it finds a match then saves it. Then 3, 4, etc… Then it comes back around and starts with the second letter and checks the first letter, then the first 2, then the first 3, etc.
Obtener los Caracteres que se Repiten en una Cadena con el lenguaje de programación Python.https://gist.github.com/Fhernd/e1ebb7c55a5b76260e257ebdd0d0de96
No Comments