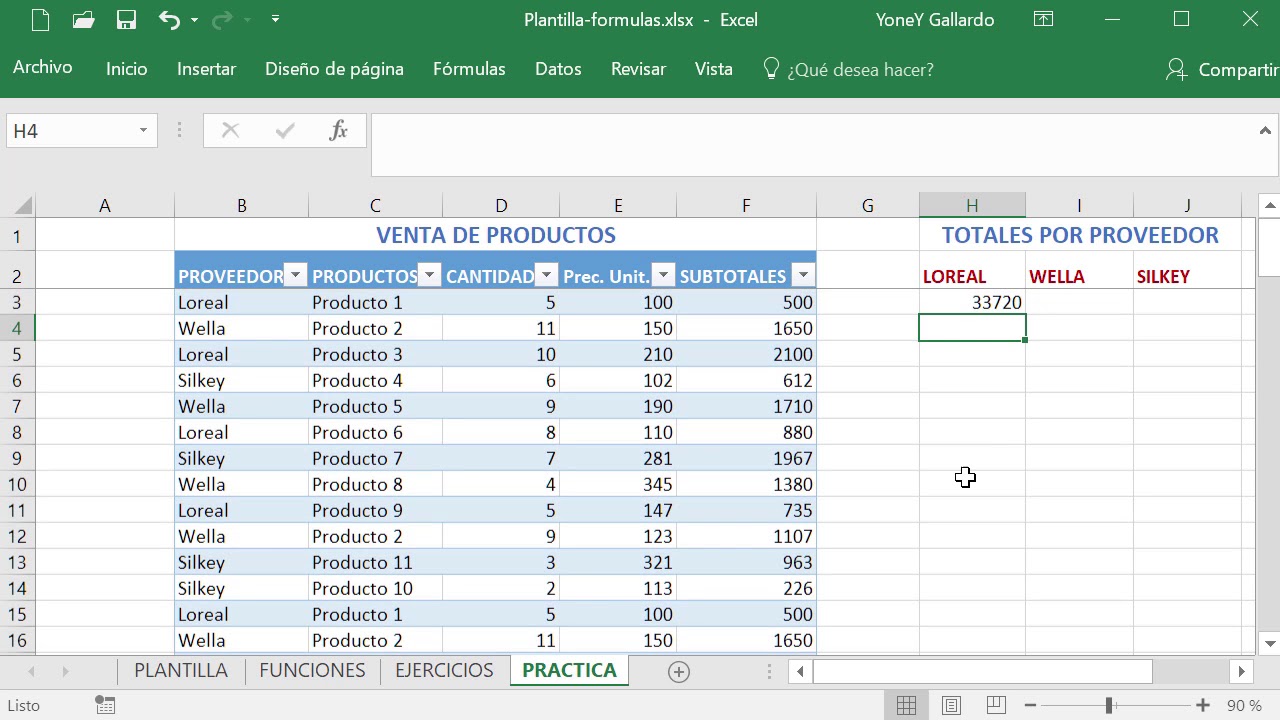
How do you sum values of objects?
Parameter breakdown:
- Q. How do you find the sum of an array of objects?
- Q. How do you add a property value to an object?
- Q. How do you add values to an array of objects?
- Q. How do you sum an array in typescript?
- Q. How do you set a property of an object Python?
- Q. How do you add two objects together?
- Q. How to sum a property value in an array?
- Q. How to sum property values of an object in JavaScript?
- Q. How to calculate the sum between two values?
- Q. How to sum cells that begin with a specific value?
- Q. How do you sum an item in JavaScript?
- Q. How do you add a property to an object in JavaScript?
- Q. How do you sum two variables in JavaScript?
- Q. How can we add a property to the object below?
- Q. How to calculate sum of values in JavaScript?
- Q. How to sum the values of an object?
- Q. Which is an example of sum array in JavaScript?
- Q. How to get all property values of a JavaScript Object?
- Q. How do you combine two objects with sum of values?
- Q. How do you find all the values of an object?
- Q. How to output all subsets that sum to a particular value?
- Q. How to use Object.keys, values, entries?
- Q. How to count number of properties in object?
- Q. How to sum the values of a JavaScript Object?
- Q. What is an object property?
- Q. How can we merge two objects dynamically?
- Q. How to add an object to a collection?
- Q. How do you add properties to an object?
- Q. How to add an element to a collection in Visual Basic?
- Q. What happens when I add b to a collection in PowerShell?
- obj = either an object or an array.
- property = the property within the nested objects/arrays you wish to perform the aggregate method on.
- aggregate = the aggregate method (sum, min, max, or count)
- shallow = can either be set to true/false or a numeric value.
Q. How do you find the sum of an array of objects?
When you want to sum the price of the items, you can use the reduce method of an array: // totalPrice is 92 let totalPrice = cart. reduce(function (accumulator, item) { return accumulator + item. price; }, 0);
Q. How do you add a property value to an object?
How to Add Property to an object in JavaScript
- var obj = { Name: “Joe” };
- obj. Age = 12;
- console. log(obj. Age)
- obj[‘Country’] = “USA”
- console. log(obj. Country)
Q. How do you add values to an array of objects?
The unshift() function is one more built-in array method of JavaScript. It is used to add the objects or elements in the array. Unlike the push() method, it adds the elements at the beginning of the array. “Note that you can add any number of elements in an array using the unshift() method.”
Q. How do you sum an array in typescript?
If we need to sum a simple array, we can use the reduce method, that executes a reducer function (that you provide) on each member of the array resulting in a single output value.
Q. How do you set a property of an object Python?
Python property() function
- fget() – used to get the value of attribute.
- fset() – used to set the value of attribute.
- fdel() – used to delete the attribute value.
- doc() – string that contains the documentation (docstring) for the attribute.
Q. How do you add two objects together?
To merge objects into a new one that has all properties of the merged objects, you have two options:
- Use a spread operator ( )
- Use the Object. assign() method.
Q. How to sum a property value in an array?
A few already gave a good answer, using reduce is the way to go here. Since most of us can already afford to use ES2015 syntax, here’s my proposition: We’re making it an immutable function while we’re at it. What reduce is doing here is simply this: Start with a value of 0 for the accumulator, and add the value of the current looped item to it.
Q. How to sum property values of an object in JavaScript?
Sum values of an object with for..in in Javascript Another interesting way to sum object values in Javascript is with for..in loop, which iterates over properties of an object. We can sum up the values with for..in by creating a variable total and adding the next value within the for..in loop to the total variable.
Q. How to calculate the sum between two values?
Using SUMIFS to Calculate the Total Between Two Values 1 Use the SUMIFS function when there are multiple criteria. 2 Select the sum_range for the SUMIFS function 3 Select the cell reference for criteria_range1. 4 Set criteria1 for the SUMIFS function. 5 Add the additional criteria to the SUMIFS function.
Q. How to sum cells that begin with a specific value?
Sum if begins with. To sum cells if other cells begin with a specific value, you can use the SUMIF function . In the example shown, the formula in G6 is: = SUMIF ( C5:C11 , “t-shirt*” , D5:D11 ) This formula sums the amounts in column D when a value in column…
Q. How do you sum an item in JavaScript?
Use the for Loop to Sum an Array in a JavaScript Array Copy const array = [1, 2, 3, 4]; let sum = 0; for (let i = 0; i < array. length; i++) { sum += array[i]; } console. log(sum); We initialize a variable sum as 0 to store the result and use the for loop to visit each element and add them to the sum of the array.
Q. How do you add a property to an object in JavaScript?
Q. How do you sum two variables in JavaScript?
Basic JavaScript Addition Add numbers in JavaScript by placing a plus sign between them. You can also use the following syntax to perform addition: var x+=y; The “+=” operator tells JavaScript to add the variable on the right side of the operator to the variable on the left.
Q. How can we add a property to the object below?
To add a new property to an object, specify the object name followed by: a dot, the name of the new property, an equals sign, and the value for the new property (enclosed in quotes if it is a string).
Q. How to calculate sum of values in JavaScript?
You can see the example the below: var sum = numArr.reduce (function(a, b) {return a+b;}) We will also use the javascript map method to the sum of array values. If you want the sum of the array values, you can use like below: Next, we will take an example of a sum array of objects using the javascript for loop method.
Q. How to sum the values of an object?
Sum values of an object with .reduce() First, get the values of each property using the Object.values()function: js Copy 1.constobj ={a:1,b:2,c:3,d:4}; 2.constvalues =object.values(obj); 3. 4.// [1,2,3,4] Object.values()returns an array of the objects property values.
Q. Which is an example of sum array in JavaScript?
Here, we will take an example of the sum array of objects using the javascript reduce method. My name is Devendra Dode. I am a full-stack developer, entrepreneur, and owner of Tutsmake.com. I like writing tutorials and tips that can help other developers.
Q. How to get all property values of a JavaScript Object?
The ECMAScript 2015 specification introduces Symbol, instances of which can be used as property names. You can use the Object.getOwnPropertySymbols to get the symbols of an object to enumerate over. The new Reflect API from ECMAScript 2015 provides Reflect.ownKeys returning a list of property names and symbols.
Q. How do you combine two objects with sum of values?
All objects (baskets) in the array merged into one with the sum of each property’s (fruit) value….
- Result object, empty.
- Loop through an array of objects using Array.
- Iterate over each object’s (basket’s) key-value pairs using Object.
- Check if the result object has a property with the name of the key.
Q. How do you find all the values of an object?
values() Method: The Object. values() method is used to return an array of the object’s own enumerable property values. The array can be looped using a for-loop to get all the values of the object.
Q. How to output all subsets that sum to a particular value?
The same site geeksforgeeks also discusses the solution to output all subsets that sum to a particular value: http://www.geeksforgeeks.org/backttracking-set-4-subset-sum/ In your case, instead of the output sets, you just need to count them. Be sure to check the optimized version in the same page, as it is an NP-complete problem.
Q. How to use Object.keys, values, entries?
Object.keys, values, entries. For plain objects, the following methods are available: Object.keys(obj) – returns an array of keys. Object.values(obj) – returns an array of values. Object.entries(obj) – returns an array of [key, value] pairs.
Q. How to count number of properties in object?
Open the solution with tests in a sandbox. Write a function count (obj) that returns the number of properties in the object: Try to make the code as short as possible. P.S. Ignore symbolic properties, count only “regular” ones. Open a sandbox with tests. Open the solution with tests in a sandbox.
Q. How to sum the values of a JavaScript Object?
Our accumulator acc, starting with a value of 0, is accumulating all looped values of our object. This has the added benefit of not depending on any internal or external variables; it’s a constant function so it won’t be accidentally overwritten… win for ES2015! Now you can make use of reduce function and get the sum.
Q. What is an object property?
Object properties are defined as a simple association between name and value. All properties have a name and value is one of the attributes linked with the property, which defines the access granted to the property. Properties refer to the collection of values which are associated with the JavaScript object.
Q. How can we merge two objects dynamically?
The spread operator allows an expression to be expanded in places where multiple elements/variables/arguments are expected. It is mostly used in a variable array where there is multiple values are expected. Since javascript objects are key value paired entities, we can merge them in to one using spread operator.
Q. How to add an object to a collection?
An object of any type that specifies the element to add to the collection. Optional. A unique String expression that specifies a key string that can be used instead of a positional index to access this new element in the collection. Optional. An expression that specifies a relative position in the collection.
Q. How do you add properties to an object?
Adding New Properties. You can add new properties to an existing object by simply giving it a value. Assume that the person object already exists – you can then give it new properties:
Q. How to add an element to a collection in Visual Basic?
Visual Basic Adds an element to a Collection object. Required. An object of any type that specifies the element to add to the collection. Optional. A unique String expression that specifies a key string that can be used instead of a positional index to access this new element in the collection. Optional.
Q. What happens when I add b to a collection in PowerShell?
If we would then add $B to the same collection the item in the collection would have an empty value in the field “fieldA” and no field “fieldC” (fieldB would be added normally to the list). Keep this in mind when adding different types of objects to a collection.
Veremos cómo podemos sumar por Categorías en Microsoft Excel
No Comments