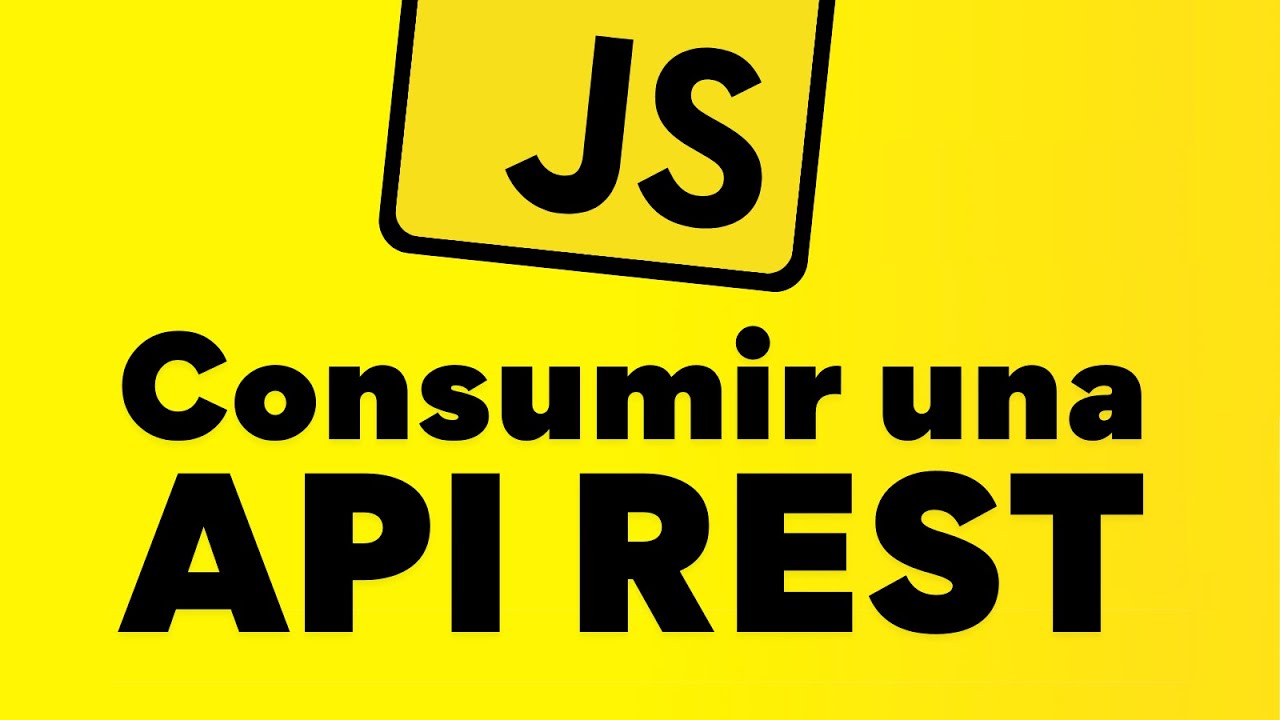
How does a promise chain handle an error?
Promise chains are great at error handling. When a promise rejects, the control jumps to the closest rejection handler. That’s very convenient in practice. For instance, in the code below the URL to fetch is wrong (no such site) and .catch handles the error: As you can see, the .catch doesn’t have to be immediate.
- Q. How are promise retry patterns used in JavaScript?
- Q. Can You Wrap setTimeout in a promise function?
- Q. How to handle errors in promises in JavaScript?
- Q. How does catch handle errors in JavaScript promises?
- Q. How to return from a promise’s catch / then block?
- Q. When do you call catch on a promise?
- Q. Can a promise chain have more than one block?
- Q. How to chain promises in JavaScript with handler?
- Q. Which is the instance method of the promise object?
- Q. How to handle promise inside loop [ codehandbook ]?
- Q. How to flatten a promise chain in JavaScript?
- Q. How to run promises in a loop sequentially?
- Q. Which is an example of promises chaining in JavaScript?
- Q. What happens when you throw a promise in JavaScript?
- Q. When to use promises in frontend programming?
- Q. What is the power of promise chaining in react?
- Q. How to chain collections in Node.js with promises?
- Q. When does fetch reject a promise in MDN?
- Q. What is the function called if the promise is rejected?
- Q. What happens if you don’t return a promise?
- Q. How to break promise chain and call function based?
- Q. Why can I not throw inside a promise?
- Q. Can a promise catch an error in node?
- Q. What happens to an exception in a promise handler?
- Q. What happens when a promise is returned in JavaScript?
- Q. What does a promise object do in MDN?
Q. How are promise retry patterns used in JavaScript?
Promise Retry Design Patterns 1 Pattern that keep on retrying until the promise resolves (with delay and maxRetries). 2 Pattern that keeps on retrying until the condition meets on the result (with delay and maxRetries). 3 A memory efficient dynamic Pattern with unlimited retries (delay provided). More
Q. Can You Wrap setTimeout in a promise function?
Luckily we can wrap setTimeout in a promise. Best practice is to wrap problematic functions at the lowest possible level, and then never call them directly again: Basically, the promise constructor takes an executor function that lets us resolve or reject a promise manually.
Q. How to handle errors in promises in JavaScript?
In non-browser environments like Node.js there are other ways to track unhandled errors. .catch handles errors in promises of all kinds: be it a reject () call, or an error thrown in a handler. We should place .catch exactly in places where we want to handle errors and know how to handle them.
Q. How does catch handle errors in JavaScript promises?
.catch handles errors in promises of all kinds: be it a reject () call, or an error thrown in a handler. We should place .catch exactly in places where we want to handle errors and know how to handle them. The handler should analyze errors (custom error classes help) and rethrow unknown ones (maybe they are programming mistakes).
Q. How to return from a promise’s catch / then block?
Returning a regular value from a reject handler, causes the next .then () resolve handler to be called (e.g. normal processing continues), Throwing in a reject handler causes normal resolve processing to stop and all resolve handlers are skipped until you get to a reject handler or the end of the chain.
Q. When do you call catch on a promise?
In the second example, you are not calling catch on the original promise, you call catch on the promise returned by then. If a then has no error callback provided, it will not stop on a rejected promise. So the promise will end in the catch.
Q. Can a promise chain have more than one block?
It’s actually really easy to achieve a good structure using Promise chains. Building the right mindset is the laborious part. All in all, here’s what I’ve learned from this quest: If you don’t have only one single .catch block in your Promise chain, you’re doing it wrong.
Q. How to chain promises in JavaScript with handler?
A handler, used in .then (handler) may create and return a promise. In that case further handlers wait until it settles, and then get its result. Here the first .then shows 1 and returns new Promise (…) in the line (*).
Q. Which is the instance method of the promise object?
The instance method of the Promise object such as then (), catch (), or finally () returns a separate promise object. Therefore, you can call the promise’s instance method on the return Promise.
Q. How to handle promise inside loop [ codehandbook ]?
Create a folder called promise_loop. Initialize the project folder using npm. Create a file called helper.js which will make use of the request module to make the API calls. Install the request module using npm. Add the following code to helper.js :
Q. How to flatten a promise chain in JavaScript?
A good rule-of-thumb is to always either return or terminate promise chains, and as soon as you get a new promise, return it immediately, to flatten things: doSomething().then(function(result) { return doSomethingElse(result); }).then(newResult => doThirdThing(newResult)).then(() => doFourthThing()).catch(error => console.error(error));
Q. How to run promises in a loop sequentially?
We wrapped the Promise into a function. This is how we can pass a parameter to it. We also changed the resolve () call to return the milliseconds value, so our .then () branch can tell us how much waiting has just been completed. The above code calls delay with a parameter of 12,000. Our script will wait 12,000 milliseconds, then say:
Q. Which is an example of promises chaining in JavaScript?
Promises chaining 1 Returning promises. A handler, used in .then (handler) may create and return a promise. 2 Example: loadScript. Here each loadScript call returns a promise, and the next .then runs when it resolves. 3 Bigger example: fetch. In frontend programming promises are often used for network requests. 4 Summary.
Q. What happens when you throw a promise in JavaScript?
If we throw inside a .then handler, that means a rejected promise, so the control jumps to the nearest error handler. new Promise((resolve, reject) => { resolve(“ok”); }).then((result) => { throw new Error(“Whoops!”); // rejects the promise }).catch(alert); // Error: Whoops! This happens for all errors, not just those caused by the throw statement.
Q. When to use promises in frontend programming?
In frontend programming promises are often used for network requests. So let’s see an extended example of that. We’ll use the fetch method to load the information about the user from the remote server. It has a lot of optional parameters covered in separate chapters, but the basic syntax is quite simple:
Q. What is the power of promise chaining in react?
This is the power of Promise chaining. By chaining Promises together, you can not only guarantee that one Promise will complete directly after the others prior to it are completed, but you can also use just a single .catch to catch any errors that may occur in all of the requests.
Q. How to chain collections in Node.js with promises?
The series of .then method calls is a chain. The first link takes the Response object from the www.7timer.info API call and executes the Body.json () method on it. The Body.json method reads the Response stream and returns a promise with a JSON object containing the data from the Response stream as its value.
Q. When does fetch reject a promise in MDN?
Per MDN, the fetch() API only rejects a promise when a “network error is encountered, although this usually means permissions issues or similar.” Basically fetch() will only reject a promise if the user is offline, or some unlikely networking error occurs, such a DNS lookup failure.
Q. What is the function called if the promise is rejected?
A Function called if the Promise is fulfilled. This function has one argument, the fulfillment value. If it is not a function, it is internally replaced with an “Identity” function (it returns the received argument). A Function called if the Promise is rejected.
Q. What happens if you don’t return a promise?
If you don’t return a promise, then wraps the return value in a resolve promise for you. This means that if you don’t return anything, you are effectively returning a resolved promise for the value undefined.
Q. How to break promise chain and call function based?
The best solution is to refactor to your promise chain to use ES6 await’s. Then you can just return from the function to skip the rest of the behavior. I have been hitting my head against this pattern for over a year and using await’s is heaven.
Q. Why can I not throw inside a promise?
As mentioned by jfriend00, the then and catch handlers are not executed synchronously. When a handler throws, it will come to an end immediately. So, the stack will be unwound and the exception would be lost. That is why throwing an exception rejects the current promise. In your case, you are rejecting inside do1 by throwing an Error object.
Q. Can a promise catch an error in node?
Yes promises swallow errors, and you can only catch them with .catch, as explained more in detail in other answers. If you are in Node.js and want to reproduce the normal throw behaviour, printing stack trace to console and exit process, you can do No, that’s not sufficient, as you would need to put that at the end of every promise chain you have.
Q. What happens to an exception in a promise handler?
Any exception in a function called as a promise fulfillment or rejection handler gets automatically converted to a rejection of the promise you’re supposed to return. The promise code that calls your function takes care of this.
Q. What happens when a promise is returned in JavaScript?
When a handler returns a value, it becomes the result of that promise, so the next .then is called with it. A classic newbie error: technically we can also add many .then to a single promise. This is not chaining. What we did here is just several handlers to one promise.
Q. What does a promise object do in MDN?
A Promise, according to MDN, object represents the eventual completion of an asynchronous operation, and its resulting value. A Promise object provides .then and .catch method, which takes in callback function to be called when the promise is resolved or rejected respectively.
Te explico cómo puedes consumir una API Rest usando Fetch. Te enseño cómo usar los headers para enviar un token y cómo puedes gestionar los errores.🔥 Puedes…
No Comments